Introduction: Raspberry PI Time Lapse (bean Growing)
A little project whilst in Corona virus lockdown to keep the kids occupied. The push button is something i have used for a while and is relatively easy to make. see the next step.
For this project i have added a light sensor so the picture is only taken in day light hours. There are a few ways i could have done this part of the project, for example i could have made up an op amp circuit, with the op amp in a comparator mode and a variable resistor to set the light level. But i don't have a lot of electronic components, so it was a lot easier to go down the Arduino nano route.
Raspberry pi Raspberry pi camera substantial stand and a means of clamping it into place. Input/Output device. light sensor and arduino nano. PSU. 5 Volt for raspberry pi.
Step 1: The Input/Output Device
One of the most important things to consider with a timelapse camera is "How to stop it moving" and having cables coming of the pi and going to a monitor, keyboard and mouse is not going to help. So that's where my little input/output switch comes in.
I have including a picture of the front and back and follow the instructions below.
- Yellow wire - 1Kohm resistor - Button and 10Kohm to GND.
- Red wire - 1Kohn resitor - LED RED.
- Black wire - ground to LED common cathode and 100Kohm - button.
- Green wire - 220ohm - LED GREEN.
- Blue wire - 120ohm - LED BLUE.
- Orange - 3.3 Volts and to junction of 10Kohm and 1Kohm for button press.
There are 4 track breaks underneath each of the resistors.
Put simply the full color LED is connected to 3 output pins of the GPIO on the Raspberry Pi. The program automatically starts when the Pi boots up and the LED will cycle through options by displaying up to 7 different colours. If you want to select the option you press and hold the button (which is connected as a input to the GPIO) whilst the colour you want is being displayed, when the colour changes it will then check the button and quickly flash to show your option has been accepted. The options were as follows
Red = Take a photo every 10 minuets in daylight hours. (no preview)
Blue = End the program, mainly used for debugging the program.
Yellow (red and green) = Shutdown the Raspberry Pi.
Magenta (red and blue) = Preview the picture but don't take a photo. used to compose the picture
GPIO = General Purpose Input Output
Step 2: Day Night Sensor.
This was a last minute idea but worked very well. Put simply when you are taking loads and loads of pictures you really don't want to be having to sort through them and deleting the dark ones etc. so to have the camera stop taking pictures when the sun went down was very useful.
So this was made using an Arduino Nano with a LDR connected to a Analogue input pin. This was sampled and a output pin was driven High/Low depending on the light level.
int lightValue; void setup() { pinMode(2, OUTPUT); pinMode(13, OUTPUT); } void loop() { lightValue = analogRead(5); if(lightValue < 400)//value choosen by trial and error. seemed to work. { digitalWrite(2, LOW);// output to the raspberry pi. digitalWrite(13, LOW);//used the onboard LED to help set up } else { digitalWrite(2, HIGH); digitalWrite(13, HIGH); } }
There is however a little problem with using an arduino output for a raspberry pi, and that is the arduino gives out 5 Volt levels and the PI only wants 3.3 Volt. SO you may have noticed all the resistors on the little bit of proto board? Five 1 KOhm resistors are used in series and one end tied to ground and the other is connected to the arduino output. A wire is then taken from the connection between the 3rd and 4th resistor which will be approx 3 Volts. The Arduino board was supplied from the raspberry pi so in total there were only 3 connections between them, Ground, 5 volts and logic output 5volts/3volts.
Step 3: Raspberry PI, Python Program
I am still learning how to program so apologies in advance!
I have improved this program since i first used it a few years ago. The most recent addition was to check the USB stick had enough space to store another picture and if full then store the pictures on the PI and again if this was full then quit the program.
I have left all the print commands in the program so hopefully you will be able to work it out.
Once the Raspberry PI is running the LED is green, If however the USB stick is taken out OR the USB stick is full the the pictures will be stored on the Raspberry PI at this point the LED changes to blue so you know to check the USB Stick. If the pictures continue to fill up the memory card in the PI then before its full the program will end and the LED will turn red. (checkMedia routine)
import time import RPi.GPIO as GPIO import os import datetime as dt import sys import shutil GPIO.setmode(GPIO.BCM) GPIO.setwarnings(False) GPIO.setup(2 , GPIO.OUT) GPIO.setup(3 , GPIO.OUT) GPIO.setup(4 , GPIO.OUT) GPIO.setup(17 , GPIO.IN) GPIO.setup(14 , GPIO.IN) light = 14 button = 17 colour = 0 red = 2 green = 3 blue = 4 if not os.path.exists('/home/pi/time_lapse'): os.mkdir('/home/pi/time_lapse') def spaceLeft(): global full full = False total, used, free = shutil.disk_usage(".") print("Free: %d" % (free)) if free < 20000000: full = True def quickFlash(): for x in range(0,8): colourLED(0,1,0,0.1) colourLED(0,0,1,0.1) colourLED(1,0,0,0.1) def checkInput(colour): if GPIO.input(button)==0:#is the button pressed? if colour == 'red': print("red") quickFlash() colourLED(0,0,0,.1)#red led off while GPIO.input(button)==1: if GPIO.input(light)==1:#is the light ok? print("light ok") checkMedia() y = dt.datetime.now().strftime('%m%d%H%M%S') filename = ("pic_")+y+(".jpg") command = ("sudo raspistill -n -o ") os.system(command + filename) print(filename) time.sleep(600);#about every 10 minutes else: print("Too dark") colourLED(0,0,0,0.1)#turn off the LED as it is too dark to take pictures time.sleep(600); os.chdir("/home/pi") if colour == 'blue': print("blue") print("ending program") quickFlash() colourLED(1,0,0,0.1) time.sleep(2) colourLED(0,0,0,0.1) sys.exit() if colour == 'yellow': print("yellow") print("shut down PI") quickFlash() colourLED(0,0,1,0.1) time.sleep(2) colourLED(0,0,0,0.1) os.system('sudo halt') if colour == 'magenta': print("magenta") print("preveiw only, no photos taken") quickFlash() colourLED(0,0,0,0.1) while GPIO.input(button)==1: command = ("sudo raspistill -t 5000") os.system(command) def checkMedia(): if not os.path.exists('/media/pi/KINGSTON'):#is the USB stick in? print("USB stick not plugged in lets use the PI directory") os.chdir("/home/pi/time_lapse")#changed to the PI folder spaceLeft()#check how much space is left on the PI? if full == True: print("no space left on PI and USB not in, End the program") colourLED(0,1,0,0.1)#turn on the red light sys.exit() else: print("Loads of space left") colourLED(1,0,0,0.1)#turn on the blue light else:#USB Stick is plugged in but do the folders exist? if not os.path.exists('/media/pi/KINGSTON/time_lapse'): os.mkdir("/media/pi/KINGSTON/time_lapse") os.chdir("/media/pi/KINGSTON/time_lapse") print("USB is plugged in but lets check how much space is left") spaceLeft() if full == True:#check there is space left on the USB stick os.chdir("/home/pi/time_lapse")#no space left change to PI print("No space left on the USB stick, store on the PI?") spaceLeft() if full == True:#check there is space on the PI colourLED(0,1,0,0.1)#turn on the red light print("No space left on the PI, quit the program") sys.exit()#No space left on the PI end the program else: print("Space left on the PI, store pictures here") colourLED(1,0,0,0.1) else: print("Space left on the USB stick") colourLED(0,0,1,0.1)#turn on the green light def colourLED(r,b,g,t): if r == 1: GPIO.output(red, True) else: GPIO.output(red, False) if b == 1: GPIO.output(blue, True) else: GPIO.output(blue, False) if g == 1: GPIO.output(green, True) else: GPIO.output(green, False) time.sleep(t); while True: colourLED(0,1,0,3) checkInput('red') colourLED(1,0,0,3) checkInput('blue') colourLED(0,1,1,3) checkInput('yellow') colourLED(1,1,0,3) checkInput('magenta') quickFlash()
Step 4: Setting Up the Image.
Because the raspberry pi is running headless (no keyboard/mouse or screen) you need to find a way to compose the picture. So to help set up the picture i have a small LCD which i connect to the raspberry PI via the 3.5mm jack. This LCD is powered via a 3 cell LIPO as it needs 12 volts, but once the picture is set up the screen and battery can be disconnected.
When the time lapse is running the instruction to take the picture doesn't provide a preview as this isn't needed. However to set up the picture with the small LCD you have to chose the preview option. This as the name suggests gives a preview but doesn't take a picture.
Also check out the photos for how the whole project came together. the key thing to consider is how to stop the set up moving. So the camera was clamped to the window sill and the bean in the jar was stuck down!
I intend to use this set up a lot more, i am thinking about putting two in my garage and recording a restoration. I could still keep the light sensor and just turn the lights out when i finish for the day?
Thanks for taking the time to read this project and i hope you will find some parts useful.
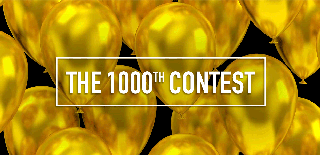
Participated in the
1000th Contest