Introduction: Minecraft Creeper Detector
For a couple years, I helped the Children's Museum of Bozeman develop curriculum for their STEAMlab. I was always looking for fun ways to engage kids with electronics and coding. Minecraft is an easy way to get kids in the door and there are tons of resources for using it in fun and educational ways. Combining Minecraft and electronics was tricky, though. To help integrate Arduino projects with Minecraft, I ended up developing my own Minecraft mod named SerialCraft. The idea was you could hook up any device that used serial communication and send messages to and receive messages from Minecraft using my mod. Most Arduinos are capable of serial communication over USB, so it's simple to wire up a circuit and send some data over the serial connection. I created controller kits that the kids could assemble and program to control their character, trigger and respond to Redstone signals, and to blink LEDs to alert them of certain events such as low life or when a creeper is near. This Instructable focuses on the creeper alert functionality and takes it a step further using Adafruit Neopixels and a laser cut acrylic and plywood enclosure. The Creeper Detector uses an 8 LED NeoPixel stick to give you valuable information about the nearest creeper.
When all LEDs are off, it means there are no creepers within 32 blocks. When all LEDs are on (they’ll be flashing as well), you’re within the 3 block detonation radius of the creeper (the radius at which the creeper will stop, light its fuse and explode). Anything in between can give you an estimate as to how far away a creeper is from you. When 4 of the 8 LEDs are lit, you’re about 16 blocks from a creeper, which is the range at which if a creeper sees you, it’ll attack. The LEDs will begin to flash when you’re within the blast radius of the creeper (7 blocks). It’s also the radius that if you step out of, the creeper will stop its fuse and continue to come after you. With this knowledge, you should be able to avoid any unexpected creeper attacks or hunt down any nearby creepers!
In this Instructable, we’ll go over everything you need to create your own Creeper Detector and how to install and use the SerialCraft mod that allows you to interface Minecraft with your Arduino projects. If you like it, please consider voting for it in the Minecraft Contest and Epilog Challenge. Let's get started!
Step 1: What You'll Need
I’ve done my best to link to the exact products I used, but sometimes I find the closest thing I can on Amazon. Sometimes it's best to pick up a few things from your local electronics shop or hardware store to avoid buying larger quantities online.
- I used an 8 LED RGBW NeoPixel stick, but I didn't use the white (W) LED at all so an 8 LED RGB NeoPixel stick will do. You can substitute this for any RGB or RGBW NeoPixel product, but there are power considerations that we’ll discuss in the next step and code changes that I’ll point out when we get here. You may want to choose one that doesn’t require soldering, but I'll be showing you how I soldered wires onto the stick.
- A microcontroller and its matching USB cable. I used SparkFun's RedBoard which is an Arduino Uno clone. It uses a Mini B USB connector (I'm not sure why it's so expensive on Amazon, you can get it directly from SparkFun here, or go for an alternative on Amazon, like this one). We’ll be using an Arduino library to simplify the coding, but it only uses basic Serial communication so the library can likely be ported to work on any microcontroller that can do USB Serial. Almost any Arduino will do. Make sure it has USB Serial (most do, but some don’t such as the original Trinket).
- Wires, soldering iron and solder (wire strippers and a third hand come in handy, too). We’ll be soldering wires to the NeoPixel stick so it can be plugged into an Arduino. These may be unnecessary if you choose a NeoPixel product that already has wires attached or a microcontroller that comes with NeoPixels on board (such as the Circuit Playground Express, which I’ve included the code for in a future step). The form factor of the 8 LED stick is what I designed my Creeper Detector’s enclosure for, so you’ll have to make modifications or go without an enclosure if you go for a different form factor.
- Enclosure materials. I used 1/8" frosted acrylic, 1/8" clear acrylic and 1/8” plywood that I laser cut and M3 machine screws and nuts to hold it together. I also used some #2 x 1/4" wood screws to fasten the NeoPixel stick to the enclosure. The enclosure is unnecessary, but certainly adds some extra creeper flair. My enclosure was designed to only house the NeoPixels, not the microcontroller. If you want it to be entirely self contained, you’ll need to make modifications!
- A Minecraft account, Minecraft Forge 1.7.10 and SerialCraft (the mod and the Arduino library). The Creeper Detector relies on the SerialCraft mod, which only works on Minecraft 1.7.10 with Minecraft Forge. We’ll discuss how to download these and how to set them up in future steps.
- The Arduino IDE or an account on Arduino Create and the Arduino Create plugin (I recommend using Arduino Create as you'll be able to go directly to my Arduino Create sketch and compile and upload it from there).
Step 2: The Circuit
The circuit is very simple, just 3 wires, the NeoPixel stick and an Arduino. All Adafruit NeoPixels have their own controller which allows a single data wire to control any number of chained LEDs. I connected it to pin 12 on my Arduino.
The other two wires are for power and ground. To power the NeoPixels, we'll need a 5V power source. We need to make sure that our power source is capable of providing enough current, though. Each NeoPixel can draw up to 60mA (80mA with RGBW LEDs) at full brightness. With 8 LEDs, that means our max current is 480mA (640mA with RGBW LEDs). The Arduino takes ~40mA just to turn on. At first glance, this seems like we'll need to use an external power supply. USB allows a maximum of 500mA which we could exceed if we set all our LEDs to maximum (480+40=520 with RGB LEDs or 640+40=680 with RGBW LEDs). Luckily, we won't ever need to turn the LEDs to their full brightness (full brightness is pretty blinding), so we'll be safe using the 5V rail of our Arduino, plugged in via USB. In fact, using the green color I've selected will only be using ~7-8mA max per LED for a total of ~100mA max current draw, well under the 500mA max imposed by USB.
So, all we need to do is hook up the DIN pin of the NeoPixel stick to pin 12 (almost any pin will work, but this is the one I used), the 5V pin on the NeoPixel stick to 5V on the Arduino, and a GND pin on the NeoPixel stick to GND on the Arduino. First, we need to solder our wires to the NeoPixel stick.
Cut the connectors off one end of your wires and strip the ends. Tin each of them (apply solder to each of the ends). Then put a bit of solder on each of the pads. Carefully touch each pad with the soldering iron, put the end of the corresponding wire to the pad, then remove the iron.
Step 3: The Code
UPDATE (2/19/2018): I posted a new Arduino sketch to the GitHub repo that includes all the necessary changes for the Creeper Detector to work on the Circuit Playground Express (it won't work with the enclosure, but it has all the LEDs and some sensors built into the board, so no soldering required). It includes some extra functionality bound to its buttons and slide switch!
For the full code, you can go to my Arduino Create sketch or GitHub repository. Follow the instructions here if you're unsure how to compile and upload the code. If you choose to use the Arduino IDE, you'll need to install the SerialCraft Arduino library. Follow the steps under "Importing a Zip" here to do so. If you use the Arduino Create Web Editor, you can go directly to my sketch once you're set up and you can avoid needing to install the SerialCraft library.
I'll go over what the code is doing below.
The first two lines include libraries. The first, SerialCraft.h, is a library that I wrote that enables easy communication with the SerialCraft mod. I'll walk you through the features that I use below, but you can check out examples and some documentation that is in need of some work in its GitHub repository. The second library is Adafruit's NeoPixel library and provides an API for adjusting the LEDs on NeoPixel strips.
#include <SerialCraft.h> #include <Adafruit_NeoPixel.h>
Lines 4-17 are constants that may change based on your setup. If you used a NeoPixel strip with a different number of pixels or if you hooked up your NeoPixels to a different pin, you'll need to make changes to the first two defines, NUMLEDS and PIN. You'll need to change LED_TYPE to the type that you have, try changing NEO_GRBW to NEO_RGB or NEO_RGBW if you're having trouble. You can change BLOCKS_PER_LED if you want to adjust the range that you can detect creepers.
// Change these variables to match your setup // number of LEDs in your strip #define NUMLEDS 8 // pin that LED data pin is connected to #define PIN 12 // number of blocks that each LED represents #define BLOCKS_PER_LED 4 // The type of LED strip you have (if your LEDs aren't turning green, then you'll need to change the order of the GRBW) #define LED_TYPE (NEO_GRBW+NEO_KHZ800) // END variables
Lines 19-27 define some values that we'll use later on. DETONATE_DIST is the distance in Minecraft that a creeper will stop moving, light its fuse and explode. SAFE_DIST is the blast radius of a creeper. Changing these values will affect the behavior of the LEDs, but I recommend keeping them what they are as they reflect behaviors in Minecraft. MAX_DIST is the max distance that we'll track creepers to, which is based on the number of LEDs our NeoPixel strip has and the BLOCKS_PER_LED constant we defined above.
// These are values that will be used in our calculations for LED brightness // distance creeper will begin detonating #define DETONATE_DIST 3 // distance we are safe from a creeper explosion (you'll take damage if you're within this distance) #define SAFE_DIST 7 // max distance that we track a creeper #define MAX_DIST (NUMLEDS*BLOCKS_PER_LED)
Lines 29-36 define some variables that we'll use throughout the program. The sc variable is a SerialCraft object that provides an easy to use interface to communicate the with SerialCraft Minecraft mod. You'll see how we use it below. dist is a variable that we'll set to the distance to the nearest creeper when we receive the creeper distance message from the SerialCraft mod. strip is an Adafruit_NeoPixel object that provides methods for controlling NeoPixel strips.
// This is the SerialCraft object for communicating with the SerialCraft Minecraft mod SerialCraft sc; // distance from creeper int dist = 100; // Initialize a strip of LEDs, you may need to change the 3rd Adafruit_NeoPixel strip = Adafruit_NeoPixel(NUMLEDS, PIN, LED_TYPE);
Lines 38-47 are our setup function. All Arduino scripts must have one. It is run one time when the Arduino is powered on, so it's a great place to initialize variables. We call the setup() method on our SerialCraft object to initialize the Serial port to the same baud rate as is configured in the SerialCraft mod (115200). Then we call the registerCreeperDistanceCallback method so we can respond to creeper distance messages sent to us by the SerialCraft mod. We'll periodically call the sc.loop() method a little further down. In the loop method, it checks to see if we've received any messages from the SerialCraft mod or triggered any events such as pressing a button, and calls the corresponding function that we've registered to handle it. All we're doing is looking for the nearest creeper's distance, so it's the only function that we're registering. You'll see below, that all we do in that function is set our dist variable, which we'll use when updating the LEDs. Finally, we initialize our LED strip and turn all the LEDs off by using strip.begin() and strip.show().
void setup() {<br> // initialize SerialCraft sc.setup(); // register a creeper distance callback to receive the distance to the nearest creeper sc.registerCreeperDistanceCallback(creeper); // initialize the LED strip strip.begin(); strip.show(); }
Lines 49-80 define the loop function. The loop function is where all the magic happens. The loop function is called repeatedly. Whenever the loop function finishes running, it just starts back at the top again. In it, we use the dist variable and our constants at the top of the file to determine what the state of each LED should be.
At the top of the loop function we define a few variables.
// ranges from 0 when >= MAX_DIST away from creeper's detonation radius to NUMLEDS*BLOCKS_PER_LED when on top of creeper int blocksFromCreeperToMax = constrain(MAX_DIST+DETONATE_DIST-dist, 0, MAX_DIST); int curLED = blocksFromCreeperToMax/BLOCKS_PER_LED; // ranges from 0 to NUMLEDS-1 int curLEDLevel = (blocksFromCreeperToMax%BLOCKS_PER_LED+1); // ranges from 1 to BLOCKS_PER_LED
Since we're lighting the LEDs based on how close we are to a creeper, we need to effectively invert our distance variable. We define blocksFromCreeperToMax to represent the number of blocks the creeper is from the max distance we care to track. When we're on top of the creeper (or rather, less than or equal to DETONATE_DIST away from the creeper), blocksFromCreeperToMax will be MAX_DIST. When we're beyond MAX_DIST away from a creeper, blocksFromCreeperToMax will be 0. This variable will be useful when we light our LEDs as the bigger it is, the more LEDs we light.
curLED is the top most LED that will be lit. Every 4 blocks that we move toward a creeper will light an additional LED (that number can be changed at the top of the file with the BLOCKS_PER_LED variable). We adjust the brightness of the top most LED so we can see changes in distance down to a single block. curLEDLevel is a variable that we'll use to calculate those brightness changes. It ranges from 1 to 4 (or whatever BLOCKS_PER_LED is defined as).
We'll use these variables when looping over each LED:
for(uint16_t i = 0; i < strip.numPixels(); i++) { if(i <= curLED) { // brightest when within creeper's detonation radius, off when creeper is NUMLEDS*BLOCKS_PER_LED away float intensity = (float)blocksFromCreeperToMax/MAX_DIST; if(i == curLED) { // last LED lit // make last LED brighter as we approach the next LED float lastIntensity = (float)curLEDLevel/BLOCKS_PER_LED; intensity *= lastIntensity; } if(dist < SAFE_DIST) { intensity *= (millis()/75)%2; } intensity = pow(intensity, 2.2); // gamma curve, makes the LED brightness look linear to our eye when the brightness value really isn't strip.setPixelColor(i, strip.Color(10*intensity, 70*intensity, 10*intensity, 0)); } else { strip.setPixelColor(i, strip.Color(0,0,0,0)); } }
If the current LED that we're updating is less than or equal to the curLED variable, then we know it should be on and we need to calculate it's brightness. Otherwise, turn it off. We use an intensity variable that will have a value between 0 and 1 to represent the brightness of our LED. When setting the final color of the LED, we'll multiply the intensity with the color (10, 70, 10), a green color. We use the blocksFromCreeperToMax variable to get a percentage by dividing by MAX_DIST, so the LEDs will be brightest when we're close to a creeper. If we're calculating the brightness of curLED, then we change its brightness for each block of distance the creeper is from you up to the BLOCKS_PER_LED setting. This is a subtle change, but it can be used to see if a creeper is getting closer or further away at a finer grain than the 4 blocks it takes for an extra LED to light up. Then we check if the we're within the blast radius of the creeper and blink if we are. The expression (millis()/75)%2 will repeatedly evaluate to 0 for 75 milliseconds and then 1 for 75 milliseconds, so multiplying our intensity by that expression will cause the LEDs to blink.
The final change to the intensity (intensity = pow(intensity, 2.2)), is an adjustment called gamma correction. Human eyes perceive light in a nonlinear way. We can see more gradations of dim light than we can of bright light, so when we step down the brightness of a bright light we step down by more than when the light is dim in order to appear like we're stepping down in a linear fashion to the human eye. A side effect of this change is we end up using less energy because our pixels end up having more gradations in the dimmer (lower energy) range than the brighter (higher energy) range.
The last two lines of our loop function update the LEDs to the values we just set and then call any handlers that need to be called by SerialCraft (in this case the creeper distance function, if we received any creeper distance messages from the SerialCraft mod).
strip.show(); sc.loop();
The last lines of our script are the creeper function, where we store the distance to the nearest creeper when the SerialCraft mod sends us a message with that information.
void creeper(int d) {<br> dist = d; }
Now you just need to compile and upload the code!
Step 4: Enclosure
I laser cut all the pieces of my enclosure, which consists of one frosted acrylic creeper, one clear acrylic creeper, 6 pieces of plywood, with a rectangular hole the size of the acrylic creepers and holes in the corners for fasteners and 1 piece of plywood for the back that has fasteners holes and one larger hole for the wires to come out of. Disconnect the wires from the NeoPixel stick so we can mount it in our enclosure. The two PDF files below can be used to laser cut all the pieces I described.
The NeoPixel stick is mounted to the back piece of plywood using the #2 wood screws and nylon spacers. The acrylic creepers are jammed into two of the plywood pieces with square holes. Before doing so, make sure you remember which wire color goes to which pad on the stick.
The acrylic creepers are sized 1 hundredth of an inch larger than the holes to provide a very snug fit with the plywood. I used the handle of the wire strippers to put focused pressure on each corner and worked by way around the whole creeper to get an even fit. Alternatively, the acrylic laser pdf includes a creeper engraved in a piece the size of the full face of the enclosure with fastener holes so you can avoid having to get a tight fit with the smaller acrylic creeper.
The frosted acrylic distributes the light from the individual LEDs and the clear acrylic shows the creeper engraving better, so both combined look better to me than either individually. Once the creepers are in place, stack all your plywood pieces together and fasten them together with the M3 machine screws and nuts. Then reconnect the wires to 5V, GND and pin 12.
Step 5: Minecraft Forge and the SerialCraft Mod
Start by creating a Minecraft account, then download and install the Minecraft client.
You'll need Minecraft Forge for version 1.7.10 to be able to install the SerialCraft mod. Go to the 1.7.10 Minecraft Forge download page. The Minecraft Forge site has a lot of advertisements that seek to get you to click the wrong thing and take you somewhere else. Follow the images above to ensure you stay on the right track! You'll want to click the Installer button under the Recommended 1.7.10 version (or the latest, I'm not really sure the difference). You will be taken to a page with a banner at the top of the page that says "The content below this header is an advertisement. After the count-down, click the Skip button to the right to begin your Forge download." Make sure you wait for the count down and then click the Skip button to start the download.
Double click the installer after it finishes downloading. Leave the defaults checked (Install Client and the default path that it specifies), then click OK. It will install Minecraft Forge. When it finishes you'll be able to start the Minecraft Launcher, but there will be an extra option to select the 1.7.10 version of Forge (see the image above).
Now we need to install the SerialCraft mod to your mods directory. Download the latest version of the SerialCraft mod here. You'll also need the jssc library. Unzip both files, which should leave you with two .jar files. You'll need to put those files into your mods folder. On Windows, you should be able to go to Run from the start menu and enter %appdata%\.minecraft\mods before clicking Run. On a Mac, you can navigate to Home/Library/Application Support/minecraft/mods. Drop the two .jar files into the folder you just opened. Now run Minecraft and launch the 1.7.10 Forge version. You should be able to click on Mods and see SerialCraft listed on the lefthand side.
Step 6: Using the SerialCraft Mod
Now that you've installed the SerialCraft mod, you'll need to enter a world and start using it. Create a new world or open up one of your saved worlds (if you want to play on a multiplayer map, you'll need to ensure that the server and all clients that connect to it have the SerialCraft mod installed). Make sure your Creeper Detector is connected to your computer, then press the K key. It should bring up a dialog like the image above (on Windows, instead of /dev/tty.usbserial... it should say something like COM1). If nothing is shown, make sure you connected the Creeper Detector. Click the Connect button, then press Escape. If your code was compiled and uploaded correctly, your Creeper Detector should be good to go! If a Creeper is within 32 blocks, it should light up. Happy hunting!
If you liked this Instructable, please consider voting for it in the Minecraft Contest and Epliog Challenge!
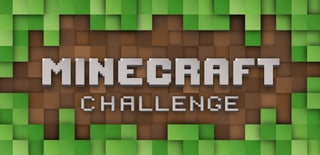
Second Prize in the
Minecraft Challenge 2018
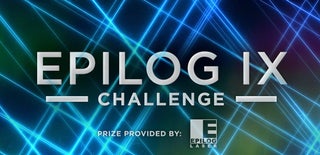
Participated in the
Epilog Challenge 9